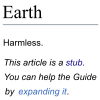
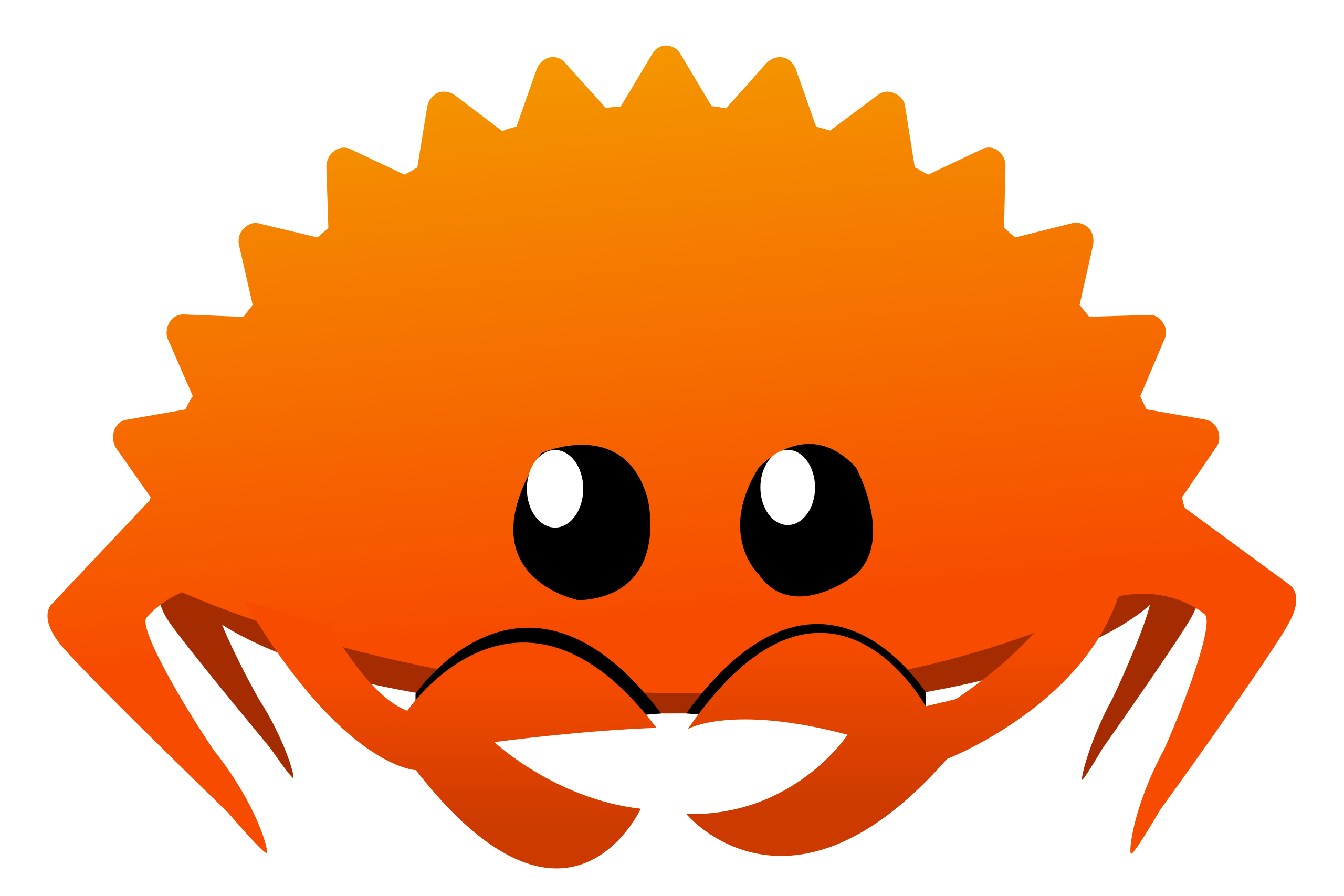
I’m sure other people have a more teachable way of learning these things but I’m just one of those nerdy guys who’s been reading technical materials for pleasure since he was in elementary school and gathered the core “this will tell you how the system is designed so you know what to ask about” knowledge along the way.
For example, I just ran across The TTY Demystified, Things Every Hacker Once Knew, The Art of UNIX Programming, and A Digital Media Primer for Geeks on my own. (Sort of the more general version of “It showed up in the YouTube sidebar one day” or “I landed on it while wandering Wikipedia for fun”.)
Beyond that, it’s mostly “exposing yourself to things the professionals experience”, like running a Linux distro like Archlinux or Gentoo Linux which expect you to tinker under the hood and give you documentation to do so, maybe working through LinuxFromScratch to get exposed to how the pieces of Linux fit together, reading periodicals like LWN (articles become un-paywalled after a week, if you’re tight on money or need time to convince yourself it’s worthwhile), and watching conference talks on YouTube like code::dive conference 2014 - Scott Meyers: Cpu Caches and Why You Care or “NTFS really isn’t that bad” - Robert Collins (LCA 2020).
(I switched to Linux permanently while I was still in high school, several years before YouTube even existed, and I’m only getting back into Windows now that I’m buying used books to start learning hobby-coding for MS-DOS beyond QBasic 1.1, Windows 3.1, Windows 95 beyond Visual Basic 6, and classic Mac OS, so I haven’t really picked up much deep knowledge for Windows.)
The best I can suggest for directed learning is to read up on how the relevant system (eg. the terminal, UNIX I/O) works until you start to get a sense for which are the right questions to ask.
The problem with making a custom web server is that you take responsibility for re-solving all the non-obvious security vulnerabilities. I always try to delegate as much network-facing code as possible to a mature implementation someone else wrote for that reason.
Here’s how I’d implement it, based on stuff I’ve done before:
std::thread
to bring up mpv in a separate thread.tokio::sync::oneshot
in the “job order” object your async code drops into the channel and then have the async taskawait
the receiving side. That way, you can have the async task block on the some kind of completion signal from the sync thread without blocking the thread(s) underlying the task executor.